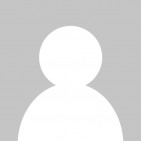
- Abstract
-
A brief set of instructions detailing the main code elements needed to check for and handle data concurrency exceptions. Such exceptions handling allows for a complex Domain Model, as wel as passing all differences and changes that have been applied to the complete domain object.
-
Introduction
Setting up your code to handle data concurrency exceptions involves using certain abstract base classes for the various domain classes and viewmodel classes. Additionally it makes use of multiple helper and extension methods to ensure data exceptions are correctly translated to the modelstates messages allowing for the messages to be correctly displayed on the UI layer, eg. web site.
The first section of this document wil deal with the infrasstructure part of the design. This constitutes the Data Access layer, or project Infrastructure. The second section deals with the UI and Application logic layer, specifically and ASP.Net MVC web application.
Background
Data Access / Infrastructure
The infrastructure deals with the Domain Model design and Data Access, in this case using Entity Framework Core (EF Core) accessing a MS SQL Server database..
Domain Model
Following the principles of Domain Driven Design (DDD), the main domain classes, representing the Entity and ValueObject, should be derived from the EntityBase and ValueObjectBase classes. These contain the necessary properties needed by the other classes and methods that process the data concurrency exceptions.
EntityBase class
The EntityBase class defines the Id of the Entity and proides the RowVersion property which is used by EF to detect data concurrency exceptions
/// <summary> /// Base class for Domain Aggregate (Entity) classes, /// supports the Id and RowVersions for concurrency /// </summary> public abstract class EntityBase { /// <summary> /// Gets or sets the Id of the Entity /// </summary> public int Id { get; set; } /// <summary> /// Gets or sets the Concurrency RowVersion /// </summary> [Timestamp] public byte[] RowVersion { get; set; } }
The key property here is the RowVersion, which represents the timestamp in the database record and is used by EF Core to detect data concurrency exceptions.
ValueObjectBase class
The ValueObjectBase class provides and arbitrary key for the object and some fields used to determine if the valueobject is being added or removed.
/// <summary> /// Base class for Domain ValueObjects /// </summary> public abstract class ValueObjectBase { /// <summary> /// Gets or sets the Id of the ValueObject /// </summary> public int Id { get; set; } #region For Concurrency Checking /// <summary> /// Indicates whether the Attachment is being added /// </summary> public bool IsAdded => this.Id == default; private bool _isRemoved; /// <summary> /// Indicates whether the Attachment is being removed /// </summary> public bool IsRemoved => _isRemoved; /// <summary> /// Sets the <see cref="IsRemoved"/> state of the attachment /// </summary> public void SetToRemoved() { _isRemoved = true; } /* * Assist with setting property errors, using the Generic version of the methods * SetValueObjectConcurrencyPropertyErrors<TValueObject> * */ /// <summary> /// Abstract method that gets the name of the default property. Default property is /// determined within the implementation of the derived class. /// </summary> /// <returns>String containing the name of the default property</returns> public abstract string GetDefaultPropertyName(); /// <summary> /// Gets the Value of the default property. Default property is /// determined within the implementation of the derived class. /// </summary> /// <returns>string value of the default property</returns> public abstract string GetDefaultPropertyValue(); /// <summary> /// Gets the name of the Identity property, defined within this ValueObjectBase class /// </summary> public string GetKeyPropertyName => nameof(this.Id); /// <summary> /// Gets the value of the Identity property, defined within this ValueObjectBase class /// </summary> public string GetKeyPropertyValue => this.Id.ToString(); #endregion }
Additionally, it is possible to define a property as the "default" property, which is used as the default message in any general error message.
Infrastructure: Data Access
All results of data persistence processing is passed
PersistenceResult Class
Repository Class
The generic repository base class provides the basic functionality. Data concurrency processing is included within any Update or Delete method. The update method below illustrates the general structure of such a method.
/// <summary> /// Updates the instance of the aggregate model. /// </summary> /// <param name="model">Aggregate being created</param> /// <param name="includes"></param> /// <returns>Returns True if the updates were successful, otherwise False</returns> public virtual PersistenceResult Update(TModel model, string[] includes = null) { var result = new PersistenceResult(model); // Checking for existence of the TModel being updated is not included within this base Repository class. It provides an OnUpdatingEvent // Where the individual EntryStates,, for example, can be set. It also includes the ability to specify a collection of property names // to be included in the object graph. We call the Get method of this repository to include the specified (navigational) properties. var dbVersion = this.Get(i => i.Id == model.Id, null, includes).FirstOrDefault(); if (dbVersion == null) { result.ErrorMessage = $"Record {model.Id} could not be found. It is possible another user has deleted it since you retrieved it."; result.Status = PersistenceStatusEnum.Missing; return result; } // Allow the retrieved record to be passed back to the derived repo, for any necessary post update processing result.SetOriginalModel(dbVersion); _db.Set<TModel>().Attach(model); _db.Entry(model).State = EntityState.Modified; // Fire the OnUpdatingEvent, if one is specified //EventHandler<OnUpdatingEventArgs<TModel>> raiseOnUpdatingEvent = DbUpdating; //if (raiseOnUpdatingEvent != null) // raiseOnUpdatingEvent(this, new OnUpdatingEventArgs<TModel>(model, dbVersion)); OnModelUpdating(new OnUpdatingEventArgs<TModel>(model, dbVersion)); // If the repository is being used within a transaction, following the applilcation UnitOfWork pattern, return if (!_callSaveChanges) return result; // No changes have been persisted try { var changesApplied = _db.SaveChanges(); // include your normal checking and successful processing. code omitted for brevity } catch (DbUpdateConcurrencyException ex) { _logger.LogError(ex, "Cannot save, changes have been made since retrieving the project."); var entry = ex.Entries.Single(); //var propertyName = nameof(TModel.RowVersion); var propertyName = "RowVersion"; var dbValues = entry.GetDatabaseValues().GetValue<byte[]>(propertyName); entry.CurrentValues[propertyName] = dbValues; result.SetPropertyErrors<TModel>(entry); } catch (Exception ex) { // Code omitted for brevity } return result; }
- References
- Tags